Java, renowned as a widely utilized programming language, offers an extensive array of operators that empower developers to execute diverse operations. Within this set, special operators are particularly noteworthy for their distinctive functionalities. These Special Operators include the ternary operator, the instanceof operator, the dot (.) operator, and the new operator. Understanding these special operators is crucial for writing efficient and effective Java code.
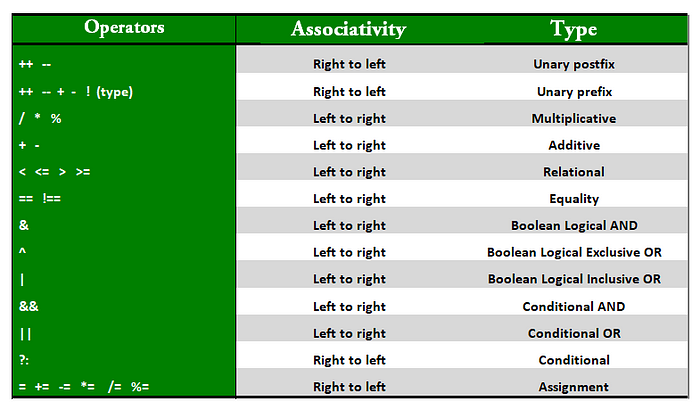
1. Ternary Operator (? :
)
The ternary operator serves as a concise alternative to the if-else statement, allowing for compact decision-making. It's also referred to as the conditional operator. The syntax of the ternary operator is as follows:
condition ? expression1 : expression2;
Here, condition
is a boolean expression. If the condition is true, expression1
is evaluated and returned; otherwise, expression2
is evaluated and returned.
Example:
int a = 10;
int b = 20;
int max = (a > b) ? a : b;
System.out.println(“The maximum value is “ + max);
In this example, the ternary operator checks if a
is greater than b
. If true, it assigns a
to max
; otherwise, it assigns b
to max
.
2. instanceof
Operator
The instanceof
operator is used to test whether an object is an instance of a specific class or a subclass thereof. This is particularly useful for type checking and downcasting in inheritance hierarchies.
Syntax:
object instanceof ClassName
3. Dot (.
) Operator
The dot operator is used to access the members of a class, such as methods, variables, and nested classes. It is one of the most commonly used operators in Java.
Example:
String s = “Hello, World!”;
int length = s.length();
System.out.println(“The length of the string is “ + length);
Here, the dot operator is used to call the length
method of the String
class to get the length of the string s
.
4. new
Operator
The new
operator is used to create new instances of objects. When the new
operator is used, it allocates memory for the object and returns a reference to that memory.
Example:
Dog myDog = new Dog();
In this example, the new
operator creates a new instance of the Dog
class and assigns it to the myDog
reference variable.
5. Array Subscript Operator ([]
)
The array subscript operator is used to access the elements of an array. It enables the retrieval or assignment of values to specific positions within the array.
Example:
int[] numbers = {1, 2, 3, 4, 5};
int firstNumber = numbers[0];
System.out.println(“The first number is “ + firstNumber);
In this example, the array subscript operator is used to access the first element of the numbers
array.
Conclusion
In Java, special operators like the ternary operator, instanceof, dot operator, new operator, and array subscript operator are invaluable for developers to craft succinct, effective, and legible code. Proficiency in these operators is crucial for Java programmers, as they enable a broad spectrum of programming activities, ranging from straightforward conditional logic to intricate type checking and object handling. Grasping and adeptly applying these operators can greatly improve one's competency in developing sturdy Java applications.
Comments
Post a Comment